What does IIFE mean in UNCLASSIFIED
IIFE (pronounced as "iffy") stands for Immediately Invoking Function Expression. It is a JavaScript coding technique that encapsulates a function and executes it immediately, isolating its scope from the global scope.
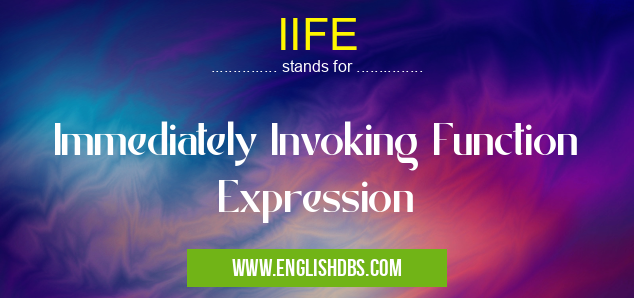
IIFE meaning in Unclassified in Miscellaneous
IIFE mostly used in an acronym Unclassified in Category Miscellaneous that means Immediately Invoking Function Expression
Shorthand: IIFE,
Full Form: Immediately Invoking Function Expression
For more information of "Immediately Invoking Function Expression", see the section below.
Introduction to IIFE
How IIFE Works
- Function Definition: An anonymous function is defined within parentheses.
- Immediate Invocation: The function is immediately invoked by adding parentheses after its definition.
- Scope Isolation: The function and its variables are enclosed within its own execution context, separate from the global scope.
Benefits of Using IIFE
- Scoped Variables: IIFEs create a new scope, preventing global variable pollution and potential conflicts.
- Code Modularity: They allow functions to be defined and executed independently, making code more modular and easier to manage.
- Data Privacy: Variables declared within an IIFE are only accessible within that function, protecting sensitive data from outside access.
- Code Execution Control: IIFEs can be used to execute code only when certain conditions are met, providing greater control over code execution.
Syntax
(function() {
// Function body
// Variables and code here are scoped to the IIFE
})();
Example
// Define and immediately execute an IIFE that logs "Hello IIFE"
(function() {
console.log("Hello IIFE");
})();
Essential Questions and Answers on Immediately Invoking Function Expression in "MISCELLANEOUS»UNFILED"
What is an IIFE?
An Immediately Invoking Function Expression (IIFE) is a JavaScript function that is defined and executed immediately upon its creation. IIFEs are typically used to create local scopes and to prevent variables and functions from polluting the global scope.
How do I create an IIFE?
There are two main ways to create an IIFE:
-
Round parentheses: Define the function within round parentheses and immediately invoke it by adding a pair of further parentheses at the end.
(function() { // Function code })();
-
Curved parentheses: Define the function using curved parentheses and immediately invoke it by prefixing it with an exclamation mark.
!function() { // Function code }();
What are the benefits of using an IIFE?
IIFEs offer several benefits, including:
- Local scope: Variables and functions defined within an IIFE are only accessible within the function, preventing them from polluting the global scope.
- Privacy: IIFEs can be used to create private variables and functions that are not accessible outside the function.
- Encapsulation: IIFEs can help to group related code and data together, making it easier to manage and maintain.
- Isolation: IIFEs can be used to isolate code from the global environment, preventing potential conflicts with other scripts or libraries.
What are the drawbacks of using an IIFE?
While IIFEs offer several benefits, there are also some potential drawbacks:
- Performance: IIFEs can have a slight performance impact, as they require the JavaScript interpreter to create a new execution context for each function invocation.
- Complexity: IIFEs can be more complex to read and understand than regular functions, especially for developers who are not familiar with the pattern.
- Debugging: Debugging IIFEs can be more challenging, as they are executed immediately upon creation, making it difficult to set breakpoints or inspect variables.
Final Words: IIFE is a versatile technique in JavaScript that allows developers to create encapsulated functions with isolated scopes. By utilizing IIFEs, developers can enhance code modularity, protect data privacy, and control code execution effectively.